Unit 7 coding: In this unit I will be exploring coding and programming. I will be learning how to code. To do this unit I will be using the software/engine called Ideone. Here I will be learning how to code and the aims and objectives for this unit is to complete the coding tasks as well the DIY tasks and doing written work and reflecting on the work/DIY task that I’ve completed, basically going through the pros and cons and problem solving.
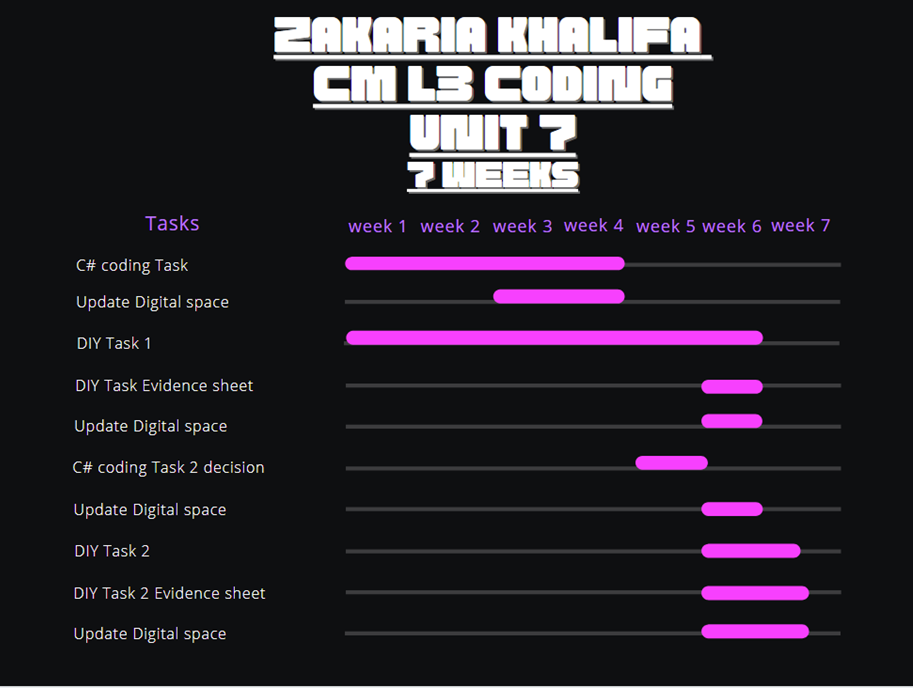
C# coding tasks 1
Task 1 Coding C#
- public class Exercise1
- {
- public static void Main( )
- {
- System.Console.WriteLine(“Hello”);
- System.Console.WriteLine(“Zakaria Khalifa”);
- }
- }
Task 2
- public class Exercise2
- {
- public static void Main()
- {
- System.Console.WriteLine(15+17);
- }
- }
Task 3
- public class Exercise3
- {
- public static void Main()
- {
- System.Console.WriteLine(36/6);
- }
- }
Task 4
- public class Exercise4
- {
- public static void Main( )
- {
- System.Console.WriteLine(-1+4*6);
- //-1 + 24 = 23
- System.Console.WriteLine((35+5)%7);
- //40 % 7 = 5 (remainder of 40/7)
- System.Console.WriteLine(14+-4*6/11);
- //14 – (24/11)= 14 – 2 = 12
- System.Console.WriteLine(2+15/6*1-7%2);
- //2 + (15/6) – remainder of (7/2) = 2 + 2 -1 = 4 – 1 = 3
- }
- }
Task 5
using System;
public class Test
{
public static void Main( )
{
// get a box of memory for soring a number
int numberBox;
// set its value
// take 22 and put it in the memory location named numberBox
numberBox=22;
// print the value on screen
// NOTE the word numberBox is not printed, its looks inside and
// print its value, in this case 22
Console.WriteLine(numberBox);
// output with text
Console.WriteLine(“The value in numberBox = {0}” ,numberBox);
//the {0} hols a space for items after the comma
//the code reads the value from from numberBox and puts it over the {0}
}
}
Task 6
- using System;
- public class Test
- {
- public static void Main( )
- {
- // get memory for soring numbers
- int numberBox;
- int numberBox2;
- // set value
- numberBox=22;
- numberBox2=44;
- // print the value on screen
- Console.WriteLine(numberBox);
- Console.WriteLine(numberBox2);
- // output with text
- Console.WriteLine(“The sum of {0} + {1} = {2}” ,numberBox, numberBox2, (numberBox+numberBox2));
- // {0} {1} {2}
- //the {0} holds a space for items after the comma
- }
- }
Task 7
- using System;
- public class Test
- {
- public static void Main( )
- {
- // get memory for soring numbers
- int numberBox;
- int numberBox2;
- // set value with keyboard input, ReadLine get input
- numberBox=Convert.ToInt32( Console.ReadLine() );
- numberBox2=Convert.ToInt32( Console.ReadLine() );
- // print the value on screen
- Console.WriteLine(numberBox);
- Console.WriteLine(numberBox2);
- // output with text
- Console.WriteLine(“The sum of {0} + {1} = {2}”,numberBox, numberBox2, (numberBox+numberBox2));
- // {0} {1} {2}
- //the {0} holds a space for items after the comma
- }
- }
Task 8
- using System;
- public class Exercise7
- {
- public static void Main()
- {
- Console.Write(“Enter a number: {0} “);
- int num1= Convert.ToInt32(Console.ReadLine());
- Console.Write(“Enter another number: {1} “);
- int num2= Convert.ToInt32(Console.ReadLine());
- Console.WriteLine(“{0} + {1} = {2}”, num1, num2, num1+num2);
- Console.WriteLine(“{0} – {1} = {2}”, num1, num2, num1+num2);
- Console.WriteLine(“{0} x {1} = {2}”, num1, num2, num1*num2);
- Console.WriteLine(“{0} / {1} = {2}”, num1, num2, num1/num2);
- Console.WriteLine(“{0} mod {1} = {2}”, num1, num2, num1%num2);
- }
- }
Task 9
- using System;
- public class Exercise11
- {
- public static void Main()
- {
- int age;
- Console.Write(“Enter your age 18 “);
- age = Convert.ToInt32(Console.ReadLine());
- Console.Write(“You look younger than {0} “,age);
- }
- }
DIY Task 1 Evidence
Task: Write C# code that displays your full name, askes what year you were born and e.g.2000 and askes for the current year e.g. 2020. The application should display your name the year born and current year and calculate and display how old you are in years. Upload slot on Moodle |
Code: using System; public class Test{public static void Main(){Console.WriteLine(“Zakaria Khalifa”);Console.WriteLine(“myBirthdate=2002”);}} |
Problems: The problem I had was that wile writing the code there was an error when I tried running the code. To fix this I had to look at the coding task that I had done and to see what I did wrong and I looked at the errors that I made, and I was missing a semi colone at the end of each bracket and it ended it being a success. |
Research: The research side of things is that there are different types of codes when It comes to programming Like the most notable ones being C#, JavaScript, ruby and C and etc. C# is the most recognizable type code because it’s mostly used for developing Microsoft apps and it is pretty much identical to another type of called Java. JavaScript however is not like Java because it’s different type of programming and it allows the developers to add interactive elements to their website. Ruby is another type of code that is used for programming and is a big supplier for apps according to (Inc 2021). Reference/Bibliography: Kim, L., 2015. 10 Most Popular Programming Languages Today. [online] Inc.com. Available at: <https://www.inc.com/larry-kim/10-most-popular-programming-languages-today.html> [Accessed 14 February 2021]. |
Evaluation: For this DIY task I had to write c# sharp code that has the data of my name and ad the year I was born. When I first approached this task, I was confused because I didn’t know what to. To fix this problem I looked at my previous coding task and see how I put the codes in. to do this I used a coding programme called Ideone, here is where you can write a c# code. While writing the code I tried running the code and there were some. To fix this I looked at them to see what the errors were, and I had forgot to put the semi colone at the end of each bracket. After I put in the semi colone I tried running the code again and it appeared to be a success. |
Decision C# coding task 2
Task 1
- using System;
- public class Test
- {
- public static void Main()
- {
- Console.WriteLine(“Zakaria Khalifa”);
- Console.Write(18);
- }
- }
Task 2
- using System;
- public class Test
- {
- public static void Main()
- {
- string yourname = “Zakaria Khalifa”;
- int yourage=18;
- Console.WriteLine(yourname);
- Console.Write(yourage);
- }
- }
Task 3
- using System;
- public class Test
- {
- public static void Main()
- {
- string yourname;
- int yourage;
- Console.WriteLine(“Zakaria Khalifa”);
- yourname= Console.ReadLine();
- Console.WriteLine(“18”);
- yourage=Convert.ToInt32(Console.ReadLine());
- Console.WriteLine(“Hi {0}”,yourname);
- Console.WriteLine(“you are {0} years old”, 18);
- }
- }
Task 4
- using System;
- public class Test
- {
- public static void Main()
- {
- int yourAge;
- Console.WriteLine(“Please enter your age”);
- yourAge=Convert.ToInt32(Console.ReadLine());
- if(yourAge==18)
- {
- Console.WriteLine(“you are {0} years old” ,18);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 5
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge==20)
{
Console.WriteLine(“you are {0} years old” ,20);
}
Console.WriteLine(“The end”);
}
}
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge==33)
{
Console.WriteLine(“you are {0} years old” ,33);
}
Console.WriteLine(“The end”);
Task 6
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=10)
{
Console.WriteLine(“you are 10 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 6
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=33)
{
Console.WriteLine(“you are 33 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 7
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=18)
{
Console.WriteLine(“you are 18 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 7
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=10)
{
Console.WriteLine(“you are 10 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 7
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=33)
{
Console.WriteLine(“you are 33 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 8
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge<=18)
{
Console.WriteLine(“you are 18 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 8
using System;
public class Test
{
public static void Main()
{
int yourAge;
Console.WriteLine(“Please enter your age”);
yourAge=Convert.ToInt32(Console.ReadLine());
if(yourAge>=18)
{
Console.WriteLine(“you are 18 or older “);
}
Console.WriteLine(“The end”);
}
}
Task 8
- using System;
- public class Test
- {
- public static void Main()
- {
- int yourAge;
- Console.WriteLine(“Please enter your age”);
- yourAge=Convert.ToInt32(Console.ReadLine());
- if(yourAge!=18)
- {
- Console.WriteLine(“you are 18 or older “);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 9
- using System;
- public class Exercise11
- {
- public static void Main()
- {
- int age;
- Console.Write(“Enter your age 18 “);
- age = Convert.ToInt32(Console.ReadLine());
- Console.Write(“You look younger than {0} “,age);
- }
- }
Task 10
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // ||= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==10 || boxNumber2==12 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 11
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // ||= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==10 || boxNumber2==15 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 11
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // ||= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==12 || boxNumber2==12 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 11
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // ||= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==11 || boxNumber2==11 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 12
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // &&= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==11 && boxNumber2==11 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 13
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // &&= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==10 && boxNumber2==15 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 13
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // &&= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==12 && boxNumber2==12 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 13
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // &&= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==11 && boxNumber2==11 )
- {
- Console.WriteLine(“test passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 14
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // &&= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==10 && boxNumber2==12 )
- {
- Console.WriteLine(“test passed”);
- }else
- {
- Console.WriteLine(“ERROR, test not passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 15
- using System;
- public class Test
- {
- public static void Main()
- {
- int boxNumber1=10, boxNumber2=12;
- // ||= or e.g. boxNumber1==10 or boxNumber2==12
- if(boxNumber1==12 || boxNumber2==15 )
- {
- Console.WriteLine(“test passed”);
- }else
- {
- Console.WriteLine(“ERROR, test not passed”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 16
- using System;
- public class Test
- {
- public static void Main()
- {
- int myAge=1;
- if(myAge<5)
- {
- Console.WriteLine(“test point 1”);
- }else if(myAge>=5 && myAge>=10)
- {
- Console.WriteLine(“test point 2”);
- }else if(myAge>10 && myAge>=16)
- {
- Console.WriteLine(“test point 3”);
- }
- Console.WriteLine(“The end”);
- }
- }
Task 17
- using System;
- public class Test
- {
- public static void Main()
- {
- int myAge=10;
- switch(myAge){
- case 1:Console.WriteLine(“test point 1”);
- break;
- case 10:Console.WriteLine(“test point 2”);
- break;
- case 16:Console.WriteLine(“test point 3”);
- break;
- case 18:Console.WriteLine(“test point 4”);
- break;
- default: Console.WriteLine(“Error”);
- break;
- }
- Console.WriteLine(“The end”);
- }
- }
DIY Task 2 Evidence
Task: Develop a C# code that uses asks for a user Age as in data type. This number is then used in 3 if() statements and tests. Upload slot on Digital space. Age is greater than 0 and less than 5, and output is “infant”Age is greater than 5 but less than or equal to 16 and outputs “school”Age is greater than 16, and outputs “college” |
Code: using System; public class Test{public static void Main(){int yourAge; Console.WriteLine(“please enter your age”);yourAge=Convert.ToInt32(Console.ReadLine()); if(yourAge>=3){ Console.WriteLine(“your are {0} years old, yourAge”);} Console.WriteLine(“The end”);}}using System; public class Test{public static void Main(){int yourAge; Console.WriteLine(“please enter your age”);yourAge=Convert.ToInt32(Console.ReadLine()); if(yourAge>=10){ Console.WriteLine(“your are {10} years old, yourAge”);} Console.WriteLine(“The end”);}} using System; public class Test { public static void Main() { int yourAge; Console.WriteLine(“please enter your age”); yourAge=Convert.ToInt32(Console.ReadLine()); if(yourAge>=16) { Console.WriteLine(“your are {18} years old, yourAge”); } Console.WriteLine(“The end”); } } |
Problems: The problem I had with this task is that I got confused on what I had to do in order to complete it was. When developing the C# code, I had trouble with the right symbols in because every time I would run the code, I would get error and I had to look at the code and figure out what and I ended put the right symbols in and tried running it again and it ended up being a success. Another problem I had with this task is that I did not know how to approach it. To fix this I had to look at my decision coding task that I did, and I had to go through every single task I completed in order to complete this task. |
Research: For the research part is that there are more than one type of codes when it comes to programming. When it comes to programming there are different levels of programming low, high and major. Low programming is where you have a lower level of abstraction as they are closer to binary and further from human language. Low level languages are harder to learn and use but offer more functionality and direct control over the computer. High level programming are basically a higher level of abstraction and is a lot easier to learn and use. major programming is basically beyond high and low because there different styles of programming and there paradigm according to (Career Karma 2021). References/bibliography: Bunch, G., 2021. Types of Coding Languages Guide | Career Karma. [online] Career Karma. Available at: <https://careerkarma.com/blog/types-of-coding-languages/> [Accessed 14 February 2021]. |
Evaluation: For this DIY task I was told to write and develop a C# sharp program that has the data that askes a user’s age that is then repeated 3 time by using an infant, school and college. The problems I had was that I did not know how to approach it and. In order to complete this DIY task, I had to do a bit of research on the different types and I also looks trough my decision coding task I completed. This worked for me because it gave me a clear understanding on what the DIY task was wanting me to do and I kind of figured it out by looking through previous coding task. After finishing of the code, I tried running the code to see if it was a success and I ended getting errors. To fix this problem I had to look at the code and to see what I was missing and I was missing symbols like the semi colone and brackets, to fix this however I put in the right symbols and tried running the code again and it ended up being a success. After finishing the code I had to do the same by putting in different numbers for age purposes. I did this because that what the DIY task required and I tried running the codes and it ended up being a success. |
Unit 7: Coding Evaluation
C# coding task 1:
For this unit I was asked to learn coding and seeing what certain coding programmes you could use. For my first task I was given a task on how write a C# code and for this I had to 9 tasks in total in order to complete it. The software/program I had to use was Ideone. This worked out well because it was easy to access and less time consuming and I could easily transfer and write the code there and also there were more than one code I could chose from, but the one code that was required was a C# sharp code. However while doing the coding task I didn’t know how to do them because I kept on getting confused. To fix this problem was that I had to look at what each task was requiring and copy each code in a different way in order to complete it. Another problem I had with the coding task was that while writing the code I tried running the code and I ended up getting a bunch of errors. This didn’t go so well because I didn’t understand what the error was and I didn’t know what to do. To fix this problem I went to show the codes to my games teacher and showed what the errors was. From this I was told that some key symbols in certain lines of the code were missing for example a curly bracket, semi colons, spelling mistakes and full stops. To fix this I checked and I put right symbols in and I tried running the code again and I ended up being a success. This helped a lot because I looked at the task for each code and most of them had curly brackets and I ended up putting a regular bracket instead of putting a curly bracket and with this I ended up putting in the right full stops and checked my spelling to make sure everything was alright. After finishing of the the coding task I had to upload the slot on digital space. The problem I had with this was that I had to put each code on a word document. I did this because it was required by my games teacher and making sure I have It saved somewhere where I can get access to, so I saved it to my USB. After putting the codes on a word a document, I couldn’t upload the file on digital space because to big. To fix this problem I had to highlight each code and copy and paste them on to my digital space in the unit 7.
DIY task 1/Evidence:
After completing the first C# code I was given another task called a DIY task. To do this task the software/program I used was Ideone. To complete this I was asked to write a C# code that has my name and birth date. The problem I had with this was that I didn’t know how to approach this. To fix this problem I looked at my C# practice code that I did and looked at that and see how I could do this task. This worked well because I had sort of a clear idea on how I can do this. Also for this DIY task I was told do some research on coding. The problem I had with this is that I couldn’t find no source material about coding. However to fix this problem I had to keep looking and I looked up different codes and I find a website where there were info about different codes. This helped a lot because it gave me a lot of information about different coding programmes and different types of codes. When writing the C# code I put my name and birthdate/ year. I did this because it was required from the DIY task. When I finished the code I tried running the code to see if it was a success and I ended up getting an error. I looked at the error to see what it was and I was missing a semi colon on of the line. I edited the code and code put in the semi colon and tried running the code again and this time it ended up being a success. After I finished the code I had to fill out a evidence sheet. To do this I copied and paste my code on to the evidence sheet and I had to fill in the research elements and the problems I had when doing the task and a evaluation. I did this because it was one of the requirements and also it helped a lot because I can talk about what problems I had and what I had to do in order to complete that task and do a bit of research with it. After I finished the evidence sheet for the first DIY task I had to upload the evidence sheet on my digital space. I couldn’t upload the file on digital space because to big, but to fix this problem I had to highlight the evidence sheet and copy and paste it on to my digital space.
Decision C# code task 2:
After completing the first DIY task and coding task. I was given another task similar to the first coding task. The software I used to complete this task was Ideone. This went well because it was similar to first coding and I found it a lot more easier to do. The issue I had with this task were that there 17 tasks to complete and some of them were repeats, but I did them anyway because it was a lot more easier to do and it was less time consuming compared to the first coding task. After finishing all of the codes I copied and paste each code on to a word document an saved it to my USB. I did this because it would be a lot more easier to access for me. After doing that I had to upload all the codes on my digital space under unit 7. I could upload the word document on digital space because the file was too big. In order to fix this problem I had to copy and paste all 17 codes to my digital space and I had to upload each code one at a time.
DIY task 2:
After finishing and uploading my coding task on digital space, I was given another DIY task. When I first approached this task It looked complicated because I didn’t know what to do it. The task required write and develop a C# code for a user age and it had to be done three times by putting in different numbers. To fix this I had to look at my previous coding task that I completed to see How I could approach this and complete. This helped me because it gave me a better idea on how to do it. I was also told do some research. This helped because it gave me more information on different levels when it comes to programming and the different types of codes you use. When doing the DIY task I kind of knew on how to do it. The software/program I used was Ideone in order to complete this task. I used this because it’s easy to access. While doing the code it ended up going well because this time I ended putting the right symbols in and I knew how to operate the code. while doing this I made sure that I didn’t make no mistake and doubled checked every thing and tried running the code and it was a success. I did another two times by putting in different numbers and tried running the codes again and it was again a success. after finishing the code I then copied and pasted it in to another evidence that I had to fill out. This helped out a lot because it gave me the chance to reflect and getting some research in there as well completing the evaluation section for the second DIY task. After finishing off the Evidence sheet for the second DIY task I copied and pasted the sheet onto my digital space on the game section under unit 7.
Gannt chart:
After I finished the second DIY task I went through the unit 7 assessment to see what I had completed and the only thing I had missing was a Gannt chart. I was asked to make a Gannt chart because it was one of the requirements for unit 7 and also I had to make one to showcase what I have completed in this unit for 6/7 weeks of the semester. To do make this Gannt chart The software/cite I used was Canva. I used this because it was required by my games teacher and it is easy to use and gain access to. While doing the Gannt chart I put down the number of weeks there were in this semester and list down the tasks I that I completed. This helped a lot because it gave me a better understanding on which week Completed. After finishing of the Gannt chart I had to upload it to my digital space on the game sections in unit 7. To do this I took a screen shot and copied and pasted it to my digital space in unit 7.
Conclusion:
In conclusion If I were to do this unit again, what I would do differently is that I would make sure that I never miss a lesson because on the second week of this term I fell a bit under the weather and couldn’t attend lesson and this led me being a bit behind on some work. Another thing I would do differently is that get the work done on time because I missed a lesson and I was a bit behind. another thing I could’ve done differently is add a bit more research because when it came to the research element when doing the two DIY tasks I could’ve added a bit more research and add more reference and source material in order for me to build my research and make it a bit more strong.